// flutter ble to esp32 ble
// main.dart -- flutter - 2.8.1
// in android/build.gradle change -- ext.kotlin_version = '1.4.32'
// in android/app/build.gradle change -- minSdkVersion 21
// tested: February 1, 2022
// change _LT_ to less than
// change _GT_ to greater than
// youtube dont like angle brackets
// formatted source code -- http://crus.in/codes/ble_flutter_esp32.txt
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import './blecontroller.dart';
void main() =_GT_ runApp(GetMaterialApp(home: Home()));
class Home extends StatelessWidget {
@override
Widget build(context) {
final BleController ble = Get.put(BleController());
return Scaffold(
appBar: AppBar(title: Text('BLE demo')),
body: Column(children:[
SizedBox(height:50),
ElevatedButton(
onPressed: ble.connect,
child: Obx(() =_GT_ Text('${ble.status.value}'))),
SizedBox(height:50),
ElevatedButton(
onPressed: ble.up,
child: Text('UP')),
SizedBox(height:50),
ElevatedButton(
onPressed: ble.down,
child: Text('DOWN')),
]),
);
}
}
// filename: blecontroller.dart
import 'package:flutter/material.dart';
import 'package:flutter_reactive_ble/flutter_reactive_ble.dart';
import 'package:get/get.dart';
import 'dart:async';
class BleController {
final frb = FlutterReactiveBle();
late StreamSubscription _LT_ConnectionStateUpdate_GT_ c;
late QualifiedCharacteristic tx;
List_LT_int_GT_plus = [0x55, 0x50]; // 'UP'
List_LT_int_GT_minus = [0x44, 0x4F, 0x57, 0x4E]; // 'DOWN'
var status = 'connect to bluetooth'.obs;
void up() async {
await frb.writeCharacteristicWithoutResponse(tx,value: plus);}
void down() async {
await frb.writeCharacteristicWithoutResponse(tx,value: minus);}
void connect() async {
status.value = 'connecting...';
c = frb.connectToDevice(id: '9C:9C:1F:C5:11:7A').listen((state){
if (state.connectionState == DeviceConnectionState.connected){
status.value = 'connected!';
tx = QualifiedCharacteristic(
serviceId: Uuid.parse("1523"),
characteristicId: Uuid.parse("1525"),
deviceId:'9C:9C:1F:C5:11:7A');}});}} # use nrf connect from playstore to find id
# esp32 connected to 2 digit 7 segment
# https://electricdollarstore.com/dig2.html
# filename: ble_test.py
from ble import BLE
import time
import bluetooth
from machine import I2C
count = 0
ble = bluetooth.BLE()
b = BLE(ble)
i2 = I2C(1, freq = 100_000) # scl=25, sda=26
def on_rx(v):
global count
if v == b'UP':
count += 1
if count _LT_ 99:
i2.writeto(0x14, bytes((2, count)))
elif v == b'DOWN':
count -= 1
if count _GT_ 0:
i2.writeto(0x14, bytes((2, count)))
b.on_write(on_rx)
while True:
time.sleep(0.5)
print(".",end="")
# filename: ble.py
import bluetooth
import random
import struct
import time
from ble_advertising import advertising_payload
from machine import Pin
from micropython import const
LED_PIN = 2
_IRQ_CENTRAL_CONNECT = const(1)
_IRQ_CENTRAL_DISCONNECT = const(2)
_IRQ_GATTS_WRITE = const(3)
_FLAG_WRITE = const(0x0008)
_SERVICE_UUID = bluetooth.UUID(0x1523)
_LED_CHAR_UUID = (bluetooth.UUID(0x1525), _FLAG_WRITE)
_LED_SERVICE = (_SERVICE_UUID, (_LED_CHAR_UUID,),)
class BLE:
def __init__(self, ble, name="ESP32"):
self._ble = ble
self._ble.active(True)
self._ble.irq(self._irq)
((self._handle,),) = self._ble.gatts_register_services((_LED_SERVICE,))
self._connections = set()
self._write_callback = None
self._payload = advertising_payload(name=name, services=[_SERVICE_UUID])
self._advertise()
def _irq(self, event, data):
if event == _IRQ_CENTRAL_CONNECT:
conn_handle, _, _ = data
self._connections.add(conn_handle)
elif event == _IRQ_CENTRAL_DISCONNECT:
conn_handle, _, _ = data
self._connections.remove(conn_handle)
self._advertise()
elif event == _IRQ_GATTS_WRITE:
conn_handle, value_handle = data
value = self._ble.gatts_read(value_handle)
if self._write_callback:
self._write_callback(value)
def on_write(self, callback):
self._write_callback = callback
def _advertise(self, interval_us=500000):
self._ble.gap_advertise(interval_us, adv_data=self._payload)
// main.dart -- flutter - 2.8.1
// in android/build.gradle change -- ext.kotlin_version = '1.4.32'
// in android/app/build.gradle change -- minSdkVersion 21
// tested: February 1, 2022
// change _LT_ to less than
// change _GT_ to greater than
// youtube dont like angle brackets
// formatted source code -- http://crus.in/codes/ble_flutter_esp32.txt
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import './blecontroller.dart';
void main() =_GT_ runApp(GetMaterialApp(home: Home()));
class Home extends StatelessWidget {
@override
Widget build(context) {
final BleController ble = Get.put(BleController());
return Scaffold(
appBar: AppBar(title: Text('BLE demo')),
body: Column(children:[
SizedBox(height:50),
ElevatedButton(
onPressed: ble.connect,
child: Obx(() =_GT_ Text('${ble.status.value}'))),
SizedBox(height:50),
ElevatedButton(
onPressed: ble.up,
child: Text('UP')),
SizedBox(height:50),
ElevatedButton(
onPressed: ble.down,
child: Text('DOWN')),
]),
);
}
}
// filename: blecontroller.dart
import 'package:flutter/material.dart';
import 'package:flutter_reactive_ble/flutter_reactive_ble.dart';
import 'package:get/get.dart';
import 'dart:async';
class BleController {
final frb = FlutterReactiveBle();
late StreamSubscription _LT_ConnectionStateUpdate_GT_ c;
late QualifiedCharacteristic tx;
List_LT_int_GT_plus = [0x55, 0x50]; // 'UP'
List_LT_int_GT_minus = [0x44, 0x4F, 0x57, 0x4E]; // 'DOWN'
var status = 'connect to bluetooth'.obs;
void up() async {
await frb.writeCharacteristicWithoutResponse(tx,value: plus);}
void down() async {
await frb.writeCharacteristicWithoutResponse(tx,value: minus);}
void connect() async {
status.value = 'connecting...';
c = frb.connectToDevice(id: '9C:9C:1F:C5:11:7A').listen((state){
if (state.connectionState == DeviceConnectionState.connected){
status.value = 'connected!';
tx = QualifiedCharacteristic(
serviceId: Uuid.parse("1523"),
characteristicId: Uuid.parse("1525"),
deviceId:'9C:9C:1F:C5:11:7A');}});}} # use nrf connect from playstore to find id
# esp32 connected to 2 digit 7 segment
# https://electricdollarstore.com/dig2.html
# filename: ble_test.py
from ble import BLE
import time
import bluetooth
from machine import I2C
count = 0
ble = bluetooth.BLE()
b = BLE(ble)
i2 = I2C(1, freq = 100_000) # scl=25, sda=26
def on_rx(v):
global count
if v == b'UP':
count += 1
if count _LT_ 99:
i2.writeto(0x14, bytes((2, count)))
elif v == b'DOWN':
count -= 1
if count _GT_ 0:
i2.writeto(0x14, bytes((2, count)))
b.on_write(on_rx)
while True:
time.sleep(0.5)
print(".",end="")
# filename: ble.py
import bluetooth
import random
import struct
import time
from ble_advertising import advertising_payload
from machine import Pin
from micropython import const
LED_PIN = 2
_IRQ_CENTRAL_CONNECT = const(1)
_IRQ_CENTRAL_DISCONNECT = const(2)
_IRQ_GATTS_WRITE = const(3)
_FLAG_WRITE = const(0x0008)
_SERVICE_UUID = bluetooth.UUID(0x1523)
_LED_CHAR_UUID = (bluetooth.UUID(0x1525), _FLAG_WRITE)
_LED_SERVICE = (_SERVICE_UUID, (_LED_CHAR_UUID,),)
class BLE:
def __init__(self, ble, name="ESP32"):
self._ble = ble
self._ble.active(True)
self._ble.irq(self._irq)
((self._handle,),) = self._ble.gatts_register_services((_LED_SERVICE,))
self._connections = set()
self._write_callback = None
self._payload = advertising_payload(name=name, services=[_SERVICE_UUID])
self._advertise()
def _irq(self, event, data):
if event == _IRQ_CENTRAL_CONNECT:
conn_handle, _, _ = data
self._connections.add(conn_handle)
elif event == _IRQ_CENTRAL_DISCONNECT:
conn_handle, _, _ = data
self._connections.remove(conn_handle)
self._advertise()
elif event == _IRQ_GATTS_WRITE:
conn_handle, value_handle = data
value = self._ble.gatts_read(value_handle)
if self._write_callback:
self._write_callback(value)
def on_write(self, callback):
self._write_callback = callback
def _advertise(self, interval_us=500000):
self._ble.gap_advertise(interval_us, adv_data=self._payload)
- Категория
- Язык программирования Dart
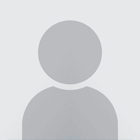
Комментариев нет.